PHP
I develop custom applications, platforms and websites with a renowned PHP Framework like Laravel or Yii2, some custom Bootstrap in Sass, in Docker containers for development.
Need a website based on a template, I'll fix it for you. I like to think along with your objective
to gain more resources out of your website. That is why I take into account not only your wishes, but also
those of your visitors.
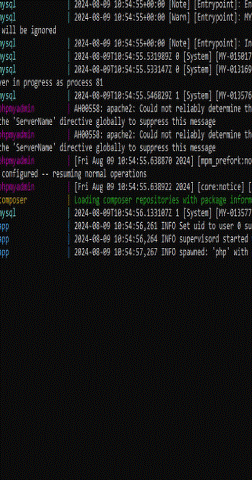
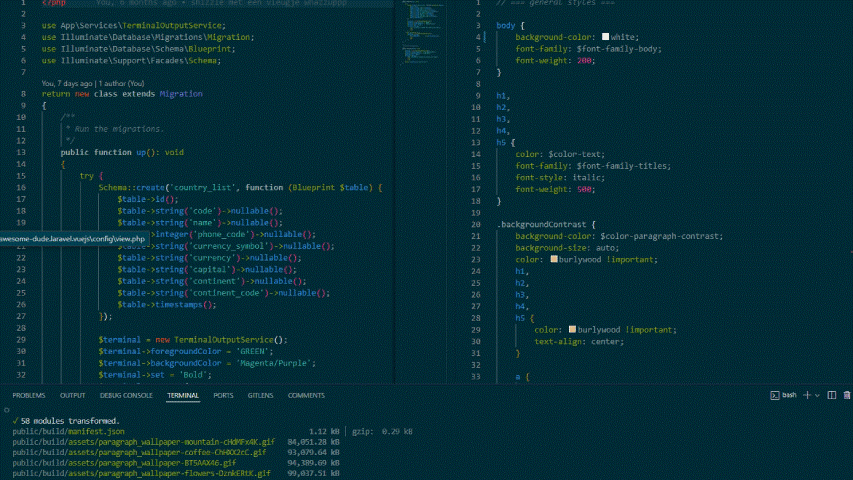
<?php
namespace App\Services;
/**
* @author Big Daddy Dudemeister
*/
class TerminalOutputService
{
/**
* @var string $backgroundColor
*/
public string $backgroundColor = 'Light Gray';
/**
* @var string $foregroundColor
*/
public string $foregroundColor = "Light Cyan";
/**
* @var string $set
*/
public string $set = 'Reset';
/**
* @access private
* @method response($text)
* @param string $text
* @return array
*/
private function response(string $text)
{
if (str_contains(strtolower($text), strtolower('Warning'))) {
return [
'response' => 'Warning',
'message' => "\033[4;91;47m" . $text . "\033[0;39;49m",
'start_code' => "\033[4;91;47m",
];
} else if (str_contains(strtolower($text), strtolower('Error'))) {
return [
'response' => 'Error',
'message' => "\033[1;91;49m" . $text . "\033[0;39;49m",
'start_code' => "\033[1;91;49m",
];
} else if (str_contains(strtolower($text), strtolower('Fatal'))) {
return [
'response' => 'Fatal',
'message' => "\033[5;31;49m" . $text . "\033[0;39;49m",
'start_code' => "\033[5;31;49m",
];
} else {
return [
'response' => 'Normal',
'message' => "\033[" . $this->settings['set'][$this->set] . ";"
. $this->settings['foreground'][$this->foregroundColor] . ";"
. $this->settings['background'][$this->backgroundColor] . "m"
. $text . "\033[0;39;49m",
'start_code' => "\033[" . $this->settings['set'][$this->set] . ";"
. $this->settings['foreground'][$this->foregroundColor] . ";"
. $this->settings['background'][$this->backgroundColor] . "m",
];
}
}
/**
* @access private
* @method compose($response)
* @param array $response
* @return string
*/
private function compose(array $response): string
{
$build = '';
foreach (explode(' ', $response['message']) as $word) {
if (array_key_exists($word, $this->settings['words'])) {
$build = $build . "\033[0;39;49m" . ' ' . "\033[" . $this->settings['set'][$this->settings['words'][$word]['set']] . ";"
. $this->settings['foreground'][$this->settings['words'][$word]['foreground']] . ";"
. $this->settings['background'][$this->settings['words'][$word]['background']] . "m"
. $word . "\033[0;39;49m" . ' '
. $response['start_code'];
} elseif ($this->isValidURL($word)) {
$build = $build . "\033[0;39;49m" . ' ' . "\033[" . $this->settings['set'][$this->settings['words']['URL']['set']] . ";"
. $this->settings['foreground'][$this->settings['words']['URL']['foreground']] . ";"
. $this->settings['background'][$this->settings['words']['URL']['background']] . "m"
. $word . "\033[0;39;49m" . ' '
. $response['start_code'];
} else {
$build = $build . $word . ' ';
}
}
return $build;
}
/**
* @access private
* @method isValidURL
* @param string $url
* @return mixed
*/
private function isValidURL($url)
{
return filter_var($url, FILTER_VALIDATE_URL);
}
/**
* @access public
* @return void Terminal Output Color tuning
* @param string $text
* @param bool $echo
* @param bool $EOL
*/
public function message(
string $text,
bool $echo = false,
bool $EOL = false
) {
$response = $this->response($text);
$composed = $this->compose($response);
$message = $composed . ($EOL ? PHP_EOL : ' ');
if ($echo) {
echo $message;
}
}
/**
* @access public
* @return array settings collection
*/
public array $settings = [
'set' => [
"Reset" => "0",
"Bold" => "1",
"Dim" => "2",
"Underlined" => "4",
"Blink" => "5",
"Reverse" => "7",
"Hidden" => "8",
"Remove Bold" => "21",
"Remove Dim" => "22",
"Remove Underline" => "24",
"Remove Blink" => "25",
"Remove Reverse" => "27",
"Remove Hidden" => "28",
],
"foreground" => [
'DEFAULT' => "39",
'BLACK' => "30",
'RED' => "31",
'GREEN' => "32",
'YELLOW' => "33",
'BLUE' => "34",
'Magenta/Purple' => "35",
'CYAN' => "36",
'WHITE' => "37",
'Dark Gray' => "90",
'Light Red' => "91",
'Light Green' => "92",
'Light Yellow' => "93",
'Light Blue' => "94",
'Light Magenta/Pink' => "95",
'Light Cyan' => "96",
'Light WHITE' => "97",
],
'background' => [
'DEFAULT' => "49",
'BLACK' => "40",
'RED' => "41",
'GREEN' => "42",
'YELLOW' => "43",
'BLUE' => "44",
'Magenta/Purple' => "45",
'CYAN' => "46",
'WHITE' => "47",
'Light Gray' => "100",
'Light Red' => "101",
'Light Green' => "102",
'Light Yellow' => "103",
'Light Blue' => "104",
'Light Magenta/Pink' => "105",
'Light Cyan' => "106",
'Light WHITE' => "107",
],
'words' => [
'created' => [
'set' => 'Blink',
'foreground' => 'Light Green',
'background' => 'DEFAULT',
],
'seed' => [
'set' => 'Bold',
'foreground' => 'Light Blue',
'background' => 'DEFAULT',
],
'dropped' => [
'set' => 'Bold',
'foreground' => 'RED',
'background' => 'DEFAULT',
],
'URL' => [
'set' => 'Blink',
'foreground' => 'Magenta/Purple',
'background' => 'Light Cyan',
]
]
];
}
<?php
namespace App\Console\Commands;
use App\Services\TerminalOutputService;
use Illuminate\Console\Command;
use Illuminate\Support\Facades\Artisan;
class MigrationCommand extends Command
{
/**
* Migration command for custom setup
*
* @var string
*/
protected $signature = 'migrate:setup {--init=fresh}';
/**
* The console command description.
*
* @var string
*/
protected $description = 'Custom migration script for setting up and maintaining the db. --init=fresh/refresh';
/**
* Execute the console command.
*/
public function handle()
{
try {
if ($this->option('init') === 'fresh') {
Artisan::call('migrate --path=database/migrations/setup');
Artisan::call('migrate --seed');
} elseif ($this->option('init') === 'refresh') {
Artisan::call('migrate:refresh --path=database/migrations/setup');
Artisan::call('migrate:refresh --seed');
}
} catch (\Exception $e) {
$message = $e->getMessage() . ' ' . $e->getTraceAsString();
(new TerminalOutputService())->message($message, true, true);
}
}
}
<?php
namespace Database\Seeders;
use App\Repositories\CountryRepository;
use App\Services\TerminalOutputService;
use Illuminate\Database\Seeder;
class CountrySeeder extends Seeder
{
/**
* Run the database seeds.
*/
public function run(): void
{
try {
$terminal = new TerminalOutputService();
$terminal->foregroundColor = 'WHITE';
$terminal->backgroundColor = 'Light Green';
$terminal->set = 'Bold';
$terminal->message(
PHP_EOL . 'Starting creating the seeds from the country seeder',
true,
true
);
$this->duplicateBackgroundsForList(new TerminalOutputService());
$countForeach = 0;
foreach ($this->country_list as $code => $country) {
$object = [
"code" => $code,
"name" => $country['name'],
"phone_code" => $country['phone'],
"currency_symbol" => $country['symbol'],
"currency" => $country['currency'],
"capital" => $country['capital'],
"continent" => $country['continent'],
"continent_code" => $country['continent_code'],
];
$countryRepository = new CountryRepository();
if ($countryRepository->checkObject($object)) {
$countryRepository->store($object);
}
$terminal = new TerminalOutputService();
$terminal->foregroundColor = 'WHITE';
$terminal->backgroundColor = $this->colorChanger($countForeach);
$terminal->set = 'Bold';
$terminal->message(
'Yes, succesfully created the seed ' . $country['name'] . ' from the country seeder',
true,
true
);
$countForeach += 1;
}
} catch (\Exception $e) {
$terminal = new TerminalOutputService();
$terminal->message(
$e->getMessage() . ' ' . $e->getTraceAsString(),
true,
true
);
}
}
private $listDuplicatedBackgrounds= [];
private function duplicateBackgroundsForList(TerminalOutputService $terminalOutputService)
{
$count = count($this->country_list);
$countBackgroundColors = count($terminalOutputService->settings['background']);
$amountSettingDuplication = round(round($count) / round($countBackgroundColors));
for ($i=0; $i < $amountSettingDuplication + 1; $i++) {
foreach ($terminalOutputService->settings['background'] as $key => $value)
{
array_push($this->listDuplicatedBackgrounds, $key);
}
}
}
private function colorChanger($countForeach)
{
return $this->listDuplicatedBackgrounds[$countForeach];
}
/**
* All countries
*/
private $country_list = array(
"AF" => array("name" => "Afghanistan", "phone" => 93, "symbol" => "؋", "capital" => "Kabul", "currency" => "AFN", "continent" => "Asia", "continent_code" => "AS"),
"AX" => array("name" => "Aland Islands", "phone" => 358, "symbol" => "€", "capital" => "Mariehamn", "currency" => "EUR", "continent" => "Europe", "continent_code" => "EU"),
"AL" => array("name" => "Albania", "phone" => 355, "symbol" => "Lek", "capital" => "Tirana", "currency" => "ALL", "continent" => "Europe", "continent_code" => "EU"),
"DZ" => array("name" => "Algeria", "phone" => 213, "symbol" => "دج", "capital" => "Algiers", "currency" => "DZD", "continent" => "Africa", "continent_code" => "AF"),
"AS" => array("name" => "American Samoa", "phone" => 1684, "symbol" => "$", "capital" => "Pago Pago", "currency" => "USD", "continent" => "Oceania", "continent_code" => "OC"),
"AD" => array("name" => "Andorra", "phone" => 376, "symbol" => "€", "capital" => "Andorra la Vella", "currency" => "EUR", "continent" => "Europe", "continent_code" => "EU"),
"AO" => array("name" => "Angola", "phone" => 244, "symbol" => "Kz", "capital" => "Luanda", "currency" => "AOA", "continent" => "Africa", "continent_code" => "AF"),
"AI" => array("name" => "Anguilla", "phone" => 1264, "symbol" => "$", "capital" => "The Valley", "currency" => "XCD", "continent" => "North America", "continent_code" => "NA"),
"AQ" => array("name" => "Antarctica", "phone" => 672, "symbol" => "$", "capital" => "Antarctica", "currency" => "AAD", "continent" => "Antarctica", "continent_code" => "AN"),
"AG" => array("name" => "Antigua and Barbuda", "phone" => 1268, "symbol" => "$", "capital" => "St. John's", "currency" => "XCD", "continent" => "North America", "continent_code" => "NA"),
"AR" => array("name" => "Argentina", "phone" => 54, "symbol" => "$", "capital" => "Buenos Aires", "currency" => "ARS", "continent" => "South America", "continent_code" => "SA"),
"AM" => array("name" => "Armenia", "phone" => 374, "symbol" => "֏", "capital" => "Yerevan", "currency" => "AMD", "continent" => "Asia", "continent_code" => "AS"),
"AW" => array("name" => "Aruba", "phone" => 297, "symbol" => "ƒ", "capital" => "Oranjestad", "currency" => "AWG", "continent" => "North America", "continent_code" => "NA"),
"AU" => array("name" => "Australia", "phone" => 61, "symbol" => "$", "capital" => "Canberra", "currency" => "AUD", "continent" => "Oceania", "continent_code" => "OC"),
"AT" => array("name" => "Austria", "phone" => 43, "symbol" => "€", "capital" => "Vienna", "currency" => "EUR", "continent" => "Europe", "continent_code" => "EU"),
"AZ" => array("name" => "Azerbaijan", "phone" => 994, "symbol" => "m", "capital" => "Baku", "currency" => "AZN", "continent" => "Asia", "continent_code" => "AS"),
"BS" => array("name" => "Bahamas", "phone" => 1242, "symbol" => "B$", "capital" => "Nassau", "currency" => "BSD", "continent" => "North America", "continent_code" => "NA"),
"BH" => array("name" => "Bahrain", "phone" => 973, "symbol" => ".د.ب", "capital" => "Manama", "currency" => "BHD", "continent" => "Asia", "continent_code" => "AS"),
"BD" => array("name" => "Bangladesh", "phone" => 880, "symbol" => "৳", "capital" => "Dhaka", "currency" => "BDT", "continent" => "Asia", "continent_code" => "AS"),
"BB" => array("name" => "Barbados", "phone" => 1246, "symbol" => "Bds$", "capital" => "Bridgetown", "currency" => "BBD", "continent" => "North America", "continent_code" => "NA"),
"BY" => array("name" => "Belarus", "phone" => 375, "symbol" => "Br", "capital" => "Minsk", "currency" => "BYN", "continent" => "Europe", "continent_code" => "EU"),
"BE" => array("name" => "Belgium", "phone" => 32, "symbol" => "€", "capital" => "Brussels", "currency" => "EUR", "continent" => "Europe", "continent_code" => "EU"),
"BZ" => array("name" => "Belize", "phone" => 501, "symbol" => "$", "capital" => "Belmopan", "currency" => "BZD", "continent" => "North America", "continent_code" => "NA"),
"BJ" => array("name" => "Benin", "phone" => 229, "symbol" => "CFA", "capital" => "Porto-Novo", "currency" => "XOF", "continent" => "Africa", "continent_code" => "AF"),
"BM" => array("name" => "Bermuda", "phone" => 1441, "symbol" => "$", "capital" => "Hamilton", "currency" => "BMD", "continent" => "North America", "continent_code" => "NA"),
"BT" => array("name" => "Bhutan", "phone" => 975, "symbol" => "Nu.", "capital" => "Thimphu", "currency" => "BTN", "continent" => "Asia", "continent_code" => "AS"),
"BO" => array("name" => "Bolivia", "phone" => 591, "symbol" => "Bs.", "capital" => "Sucre", "currency" => "BOB", "continent" => "South America", "continent_code" => "SA"),
"BQ" => array("name" => "Bonaire, Sint Eustatius and Saba", "phone" => 599, "symbol" => "$", "capital" => "Kralendijk", "currency" => "USD", "continent" => "North America", "continent_code" => "NA"),
"BA" => array("name" => "Bosnia and Herzegovina", "phone" => 387, "symbol" => "KM", "capital" => "Sarajevo", "currency" => "BAM", "continent" => "Europe", "continent_code" => "EU"),
"BW" => array("name" => "Botswana", "phone" => 267, "symbol" => "P", "capital" => "Gaborone", "currency" => "BWP", "continent" => "Africa", "continent_code" => "AF"),
"BV" => array("name" => "Bouvet Island", "phone" => 55, "symbol" => "kr", "capital" => "", "currency" => "NOK", "continent" => "Antarctica", "continent_code" => "AN"),
"BR" => array("name" => "Brazil", "phone" => 55, "symbol" => "R$", "capital" => "Brasilia", "currency" => "BRL", "continent" => "South America", "continent_code" => "SA"),
"IO" => array("name" => "British Indian Ocean Territory", "phone" => 246, "symbol" => "$", "capital" => "Diego Garcia", "currency" => "USD", "continent" => "Asia", "continent_code" => "AS"),
"BN" => array("name" => "Brunei Darussalam", "phone" => 673, "symbol" => "B$", "capital" => "Bandar Seri Begawan", "currency" => "BND", "continent" => "Asia", "continent_code" => "AS"),
"BG" => array("name" => "Bulgaria", "phone" => 359, "symbol" => "Лв.", "capital" => "Sofia", "currency" => "BGN", "continent" => "Europe", "continent_code" => "EU"),
"BF" => array("name" => "Burkina Faso", "phone" => 226, "symbol" => "CFA", "capital" => "Ouagadougou", "currency" => "XOF", "continent" => "Africa", "continent_code" => "AF"),
"BI" => array("name" => "Burundi", "phone" => 257, "symbol" => "FBu", "capital" => "Bujumbura", "currency" => "BIF", "continent" => "Africa", "continent_code" => "AF"),
"KH" => array("name" => "Cambodia", "phone" => 855, "symbol" => "KHR", "capital" => "Phnom Penh", "currency" => "KHR", "continent" => "Asia", "continent_code" => "AS"),
"CM" => array("name" => "Cameroon", "phone" => 237, "symbol" => "FCFA", "capital" => "Yaounde", "currency" => "XAF", "continent" => "Africa", "continent_code" => "AF"),
"CA" => array("name" => "Canada", "phone" => 1, "symbol" => "$", "capital" => "Ottawa", "currency" => "CAD", "continent" => "North America", "continent_code" => "NA"),
"CV" => array("name" => "Cape Verde", "phone" => 238, "symbol" => "$", "capital" => "Praia", "currency" => "CVE", "continent" => "Africa", "continent_code" => "AF"),
"KY" => array("name" => "Cayman Islands", "phone" => 1345, "symbol" => "$", "capital" => "George Town", "currency" => "KYD", "continent" => "North America", "continent_code" => "NA"),
"CF" => array("name" => "Central African Republic", "phone" => 236, "symbol" => "FCFA", "capital" => "Bangui", "currency" => "XAF", "continent" => "Africa", "continent_code" => "AF"),
"TD" => array("name" => "Chad", "phone" => 235, "symbol" => "FCFA", "capital" => "N'Djamena", "currency" => "XAF", "continent" => "Africa", "continent_code" => "AF"),
"CL" => array("name" => "Chile", "phone" => 56, "symbol" => "$", "capital" => "Santiago", "currency" => "CLP", "continent" => "South America", "continent_code" => "SA"),
"CN" => array("name" => "China", "phone" => 86, "symbol" => "¥", "capital" => "Beijing", "currency" => "CNY", "continent" => "Asia", "continent_code" => "AS"),
"CX" => array("name" => "Christmas Island", "phone" => 61, "symbol" => "$", "capital" => "Flying Fish Cove", "currency" => "AUD", "continent" => "Asia", "continent_code" => "AS"),
"CC" => array("name" => "Cocos (Keeling) Islands", "phone" => 672, "symbol" => "$", "capital" => "West Island", "currency" => "AUD", "continent" => "Asia", "continent_code" => "AS"),
"CO" => array("name" => "Colombia", "phone" => 57, "symbol" => "$", "capital" => "Bogota", "currency" => "COP", "continent" => "South America", "continent_code" => "SA"),
"KM" => array("name" => "Comoros", "phone" => 269, "symbol" => "CF", "capital" => "Moroni", "currency" => "KMF", "continent" => "Africa", "continent_code" => "AF"),
"CG" => array("name" => "Congo", "phone" => 242, "symbol" => "FC", "capital" => "Brazzaville", "currency" => "XAF", "continent" => "Africa", "continent_code" => "AF"),
"CD" => array("name" => "Congo, Democratic Republic of the Congo", "phone" => 242, "symbol" => "FC", "capital" => "Kinshasa", "currency" => "CDF", "continent" => "Africa", "continent_code" => "AF"),
"CK" => array("name" => "Cook Islands", "phone" => 682, "symbol" => "$", "capital" => "Avarua", "currency" => "NZD", "continent" => "Oceania", "continent_code" => "OC"),
"CR" => array("name" => "Costa Rica", "phone" => 506, "symbol" => "₡", "capital" => "San Jose", "currency" => "CRC", "continent" => "North America", "continent_code" => "NA"),
"CI" => array("name" => "Cote D'Ivoire", "phone" => 225, "symbol" => "CFA", "capital" => "Yamoussoukro", "currency" => "XOF", "continent" => "Africa", "continent_code" => "AF"),
"HR" => array("name" => "Croatia", "phone" => 385, "symbol" => "kn", "capital" => "Zagreb", "currency" => "HRK", "continent" => "Europe", "continent_code" => "EU"),
"CU" => array("name" => "Cuba", "phone" => 53, "symbol" => "$", "capital" => "Havana", "currency" => "CUP", "continent" => "North America", "continent_code" => "NA"),
"CW" => array("name" => "Curacao", "phone" => 599, "symbol" => "ƒ", "capital" => "Willemstad", "currency" => "ANG", "continent" => "North America", "continent_code" => "NA"),
"CY" => array("name" => "Cyprus", "phone" => 357, "symbol" => "€", "capital" => "Nicosia", "currency" => "EUR", "continent" => "Asia", "continent_code" => "AS"),
"CZ" => array("name" => "Czech Republic", "phone" => 420, "symbol" => "Kč", "capital" => "Prague", "currency" => "CZK", "continent" => "Europe", "continent_code" => "EU"),
"DK" => array("name" => "Denmark", "phone" => 45, "symbol" => "Kr.", "capital" => "Copenhagen", "currency" => "DKK", "continent" => "Europe", "continent_code" => "EU"),
"DJ" => array("name" => "Djibouti", "phone" => 253, "symbol" => "Fdj", "capital" => "Djibouti", "currency" => "DJF", "continent" => "Africa", "continent_code" => "AF"),
"DM" => array("name" => "Dominica", "phone" => 1767, "symbol" => "$", "capital" => "Roseau", "currency" => "XCD", "continent" => "North America", "continent_code" => "NA"),
"DO" => array("name" => "Dominican Republic", "phone" => 1809, "symbol" => "$", "capital" => "Santo Domingo", "currency" => "DOP", "continent" => "North America", "continent_code" => "NA"),
"EC" => array("name" => "Ecuador", "phone" => 593, "symbol" => "$", "capital" => "Quito", "currency" => "USD", "continent" => "South America", "continent_code" => "SA"),
"EG" => array("name" => "Egypt", "phone" => 20, "symbol" => "ج.م", "capital" => "Cairo", "currency" => "EGP", "continent" => "Africa", "continent_code" => "AF"),
"SV" => array("name" => "El Salvador", "phone" => 503, "symbol" => "$", "capital" => "San Salvador", "currency" => "USD", "continent" => "North America", "continent_code" => "NA"),
"GQ" => array("name" => "Equatorial Guinea", "phone" => 240, "symbol" => "FCFA", "capital" => "Malabo", "currency" => "XAF", "continent" => "Africa", "continent_code" => "AF"),
"ER" => array("name" => "Eritrea", "phone" => 291, "symbol" => "Nfk", "capital" => "Asmara", "currency" => "ERN", "continent" => "Africa", "continent_code" => "AF"),
"EE" => array("name" => "Estonia", "phone" => 372, "symbol" => "€", "capital" => "Tallinn", "currency" => "EUR", "continent" => "Europe", "continent_code" => "EU"),
"ET" => array("name" => "Ethiopia", "phone" => 251, "symbol" => "Nkf", "capital" => "Addis Ababa", "currency" => "ETB", "continent" => "Africa", "continent_code" => "AF"),
"FK" => array("name" => "Falkland Islands (Malvinas)", "phone" => 500, "symbol" => "£", "capital" => "Stanley", "currency" => "FKP", "continent" => "South America", "continent_code" => "SA"),
"FO" => array("name" => "Faroe Islands", "phone" => 298, "symbol" => "Kr.", "capital" => "Torshavn", "currency" => "DKK", "continent" => "Europe", "continent_code" => "EU"),
"FJ" => array("name" => "Fiji", "phone" => 679, "symbol" => "FJ$", "capital" => "Suva", "currency" => "FJD", "continent" => "Oceania", "continent_code" => "OC"),
"FI" => array("name" => "Finland", "phone" => 358, "symbol" => "€", "capital" => "Helsinki", "currency" => "EUR", "continent" => "Europe", "continent_code" => "EU"),
"FR" => array("name" => "France", "phone" => 33, "symbol" => "€", "capital" => "Paris", "currency" => "EUR", "continent" => "Europe", "continent_code" => "EU"),
"GF" => array("name" => "French Guiana", "phone" => 594, "symbol" => "€", "capital" => "Cayenne", "currency" => "EUR", "continent" => "South America", "continent_code" => "SA"),
"PF" => array("name" => "French Polynesia", "phone" => 689, "symbol" => "₣", "capital" => "Papeete", "currency" => "XPF", "continent" => "Oceania", "continent_code" => "OC"),
"TF" => array("name" => "French Southern Territories", "phone" => 262, "symbol" => "€", "capital" => "Port-aux-Francais", "currency" => "EUR", "continent" => "Antarctica", "continent_code" => "AN"),
"GA" => array("name" => "Gabon", "phone" => 241, "symbol" => "FCFA", "capital" => "Libreville", "currency" => "XAF", "continent" => "Africa", "continent_code" => "AF"),
"GM" => array("name" => "Gambia", "phone" => 220, "symbol" => "D", "capital" => "Banjul", "currency" => "GMD", "continent" => "Africa", "continent_code" => "AF"),
"GE" => array("name" => "Georgia", "phone" => 995, "symbol" => "ლ", "capital" => "Tbilisi", "currency" => "GEL", "continent" => "Asia", "continent_code" => "AS"),
"DE" => array("name" => "Germany", "phone" => 49, "symbol" => "€", "capital" => "Berlin", "currency" => "EUR", "continent" => "Europe", "continent_code" => "EU"),
"GH" => array("name" => "Ghana", "phone" => 233, "symbol" => "GH₵", "capital" => "Accra", "currency" => "GHS", "continent" => "Africa", "continent_code" => "AF"),
"GI" => array("name" => "Gibraltar", "phone" => 350, "symbol" => "£", "capital" => "Gibraltar", "currency" => "GIP", "continent" => "Europe", "continent_code" => "EU"),
"GR" => array("name" => "Greece", "phone" => 30, "symbol" => "€", "capital" => "Athens", "currency" => "EUR", "continent" => "Europe", "continent_code" => "EU"),
"GL" => array("name" => "Greenland", "phone" => 299, "symbol" => "Kr.", "capital" => "Nuuk", "currency" => "DKK", "continent" => "North America", "continent_code" => "NA"),
"GD" => array("name" => "Grenada", "phone" => 1473, "symbol" => "$", "capital" => "St. George's", "currency" => "XCD", "continent" => "North America", "continent_code" => "NA"),
"GP" => array("name" => "Guadeloupe", "phone" => 590, "symbol" => "€", "capital" => "Basse-Terre", "currency" => "EUR", "continent" => "North America", "continent_code" => "NA"),
"GU" => array("name" => "Guam", "phone" => 1671, "symbol" => "$", "capital" => "Hagatna", "currency" => "USD", "continent" => "Oceania", "continent_code" => "OC"),
"GT" => array("name" => "Guatemala", "phone" => 502, "symbol" => "Q", "capital" => "Guatemala City", "currency" => "GTQ", "continent" => "North America", "continent_code" => "NA"),
"GG" => array("name" => "Guernsey", "phone" => 44, "symbol" => "£", "capital" => "St Peter Port", "currency" => "GBP", "continent" => "Europe", "continent_code" => "EU"),
"GN" => array("name" => "Guinea", "phone" => 224, "symbol" => "FG", "capital" => "Conakry", "currency" => "GNF", "continent" => "Africa", "continent_code" => "AF"),
"GW" => array("name" => "Guinea-Bissau", "phone" => 245, "symbol" => "CFA", "capital" => "Bissau", "currency" => "XOF", "continent" => "Africa", "continent_code" => "AF"),
"GY" => array("name" => "Guyana", "phone" => 592, "symbol" => "$", "capital" => "Georgetown", "currency" => "GYD", "continent" => "South America", "continent_code" => "SA"),
"HT" => array("name" => "Haiti", "phone" => 509, "symbol" => "G", "capital" => "Port-au-Prince", "currency" => "HTG", "continent" => "North America", "continent_code" => "NA"),
"HM" => array("name" => "Heard Island and McDonald Islands", "phone" => 0, "symbol" => "$", "capital" => "", "currency" => "AUD", "continent" => "Antarctica", "continent_code" => "AN"),
"VA" => array("name" => "Holy See (Vatican City State)", "phone" => 39, "symbol" => "€", "capital" => "Vatican City", "currency" => "EUR", "continent" => "Europe", "continent_code" => "EU"),
"HN" => array("name" => "Honduras", "phone" => 504, "symbol" => "L", "capital" => "Tegucigalpa", "currency" => "HNL", "continent" => "North America", "continent_code" => "NA"),
"HK" => array("name" => "Hong Kong", "phone" => 852, "symbol" => "$", "capital" => "Hong Kong", "currency" => "HKD", "continent" => "Asia", "continent_code" => "AS"),
"HU" => array("name" => "Hungary", "phone" => 36, "symbol" => "Ft", "capital" => "Budapest", "currency" => "HUF", "continent" => "Europe", "continent_code" => "EU"),
"IS" => array("name" => "Iceland", "phone" => 354, "symbol" => "kr", "capital" => "Reykjavik", "currency" => "ISK", "continent" => "Europe", "continent_code" => "EU"),
"IN" => array("name" => "India", "phone" => 91, "symbol" => "₹", "capital" => "New Delhi", "currency" => "INR", "continent" => "Asia", "continent_code" => "AS"),
"ID" => array("name" => "Indonesia", "phone" => 62, "symbol" => "Rp", "capital" => "Jakarta", "currency" => "IDR", "continent" => "Asia", "continent_code" => "AS"),
"IR" => array("name" => "Iran, Islamic Republic of", "phone" => 98, "symbol" => "﷼", "capital" => "Tehran", "currency" => "IRR", "continent" => "Asia", "continent_code" => "AS"),
"IQ" => array("name" => "Iraq", "phone" => 964, "symbol" => "د.ع", "capital" => "Baghdad", "currency" => "IQD", "continent" => "Asia", "continent_code" => "AS"),
"IE" => array("name" => "Ireland", "phone" => 353, "symbol" => "€", "capital" => "Dublin", "currency" => "EUR", "continent" => "Europe", "continent_code" => "EU"),
"IM" => array("name" => "Isle of Man", "phone" => 44, "symbol" => "£", "capital" => "Douglas, Isle of Man", "currency" => "GBP", "continent" => "Europe", "continent_code" => "EU"),
"IL" => array("name" => "Israel", "phone" => 972, "symbol" => "₪", "capital" => "Jerusalem", "currency" => "ILS", "continent" => "Asia", "continent_code" => "AS"),
"IT" => array("name" => "Italy", "phone" => 39, "symbol" => "€", "capital" => "Rome", "currency" => "EUR", "continent" => "Europe", "continent_code" => "EU"),
"JM" => array("name" => "Jamaica", "phone" => 1876, "symbol" => "J$", "capital" => "Kingston", "currency" => "JMD", "continent" => "North America", "continent_code" => "NA"),
"JP" => array("name" => "Japan", "phone" => 81, "symbol" => "¥", "capital" => "Tokyo", "currency" => "JPY", "continent" => "Asia", "continent_code" => "AS"),
"JE" => array("name" => "Jersey", "phone" => 44, "symbol" => "£", "capital" => "Saint Helier", "currency" => "GBP", "continent" => "Europe", "continent_code" => "EU"),
"JO" => array("name" => "Jordan", "phone" => 962, "symbol" => "ا.د", "capital" => "Amman", "currency" => "JOD", "continent" => "Asia", "continent_code" => "AS"),
"KZ" => array("name" => "Kazakhstan", "phone" => 7, "symbol" => "лв", "capital" => "Astana", "currency" => "KZT", "continent" => "Asia", "continent_code" => "AS"),
"KE" => array("name" => "Kenya", "phone" => 254, "symbol" => "KSh", "capital" => "Nairobi", "currency" => "KES", "continent" => "Africa", "continent_code" => "AF"),
"KI" => array("name" => "Kiribati", "phone" => 686, "symbol" => "$", "capital" => "Tarawa", "currency" => "AUD", "continent" => "Oceania", "continent_code" => "OC"),
"KP" => array("name" => "Korea, Democratic People's Republic of", "phone" => 850, "symbol" => "₩", "capital" => "Pyongyang", "currency" => "KPW", "continent" => "Asia", "continent_code" => "AS"),
"KR" => array("name" => "Korea, Republic of", "phone" => 82, "symbol" => "₩", "capital" => "Seoul", "currency" => "KRW", "continent" => "Asia", "continent_code" => "AS"),
"XK" => array("name" => "Kosovo", "phone" => 383, "symbol" => "€", "capital" => "Pristina", "currency" => "EUR", "continent" => "Europe", "continent_code" => "EU"),
"KW" => array("name" => "Kuwait", "phone" => 965, "symbol" => "ك.د", "capital" => "Kuwait City", "currency" => "KWD", "continent" => "Asia", "continent_code" => "AS"),
"KG" => array("name" => "Kyrgyzstan", "phone" => 996, "symbol" => "лв", "capital" => "Bishkek", "currency" => "KGS", "continent" => "Asia", "continent_code" => "AS"),
"LA" => array("name" => "Lao People's Democratic Republic", "phone" => 856, "symbol" => "₭", "capital" => "Vientiane", "currency" => "LAK", "continent" => "Asia", "continent_code" => "AS"),
"LV" => array("name" => "Latvia", "phone" => 371, "symbol" => "€", "capital" => "Riga", "currency" => "EUR", "continent" => "Europe", "continent_code" => "EU"),
"LB" => array("name" => "Lebanon", "phone" => 961, "symbol" => "£", "capital" => "Beirut", "currency" => "LBP", "continent" => "Asia", "continent_code" => "AS"),
"LS" => array("name" => "Lesotho", "phone" => 266, "symbol" => "L", "capital" => "Maseru", "currency" => "LSL", "continent" => "Africa", "continent_code" => "AF"),
"LR" => array("name" => "Liberia", "phone" => 231, "symbol" => "$", "capital" => "Monrovia", "currency" => "LRD", "continent" => "Africa", "continent_code" => "AF"),
"LY" => array("name" => "Libyan Arab Jamahiriya", "phone" => 218, "symbol" => "د.ل", "capital" => "Tripolis", "currency" => "LYD", "continent" => "Africa", "continent_code" => "AF"),
"LI" => array("name" => "Liechtenstein", "phone" => 423, "symbol" => "CHf", "capital" => "Vaduz", "currency" => "CHF", "continent" => "Europe", "continent_code" => "EU"),
"LT" => array("name" => "Lithuania", "phone" => 370, "symbol" => "€", "capital" => "Vilnius", "currency" => "EUR", "continent" => "Europe", "continent_code" => "EU"),
"LU" => array("name" => "Luxembourg", "phone" => 352, "symbol" => "€", "capital" => "Luxembourg", "currency" => "EUR", "continent" => "Europe", "continent_code" => "EU"),
"MO" => array("name" => "Macao", "phone" => 853, "symbol" => "$", "capital" => "Macao", "currency" => "MOP", "continent" => "Asia", "continent_code" => "AS"),
"MK" => array("name" => "Macedonia, the Former Yugoslav Republic of", "phone" => 389, "symbol" => "ден", "capital" => "Skopje", "currency" => "MKD", "continent" => "Europe", "continent_code" => "EU"),
"MG" => array("name" => "Madagascar", "phone" => 261, "symbol" => "Ar", "capital" => "Antananarivo", "currency" => "MGA", "continent" => "Africa", "continent_code" => "AF"),
"MW" => array("name" => "Malawi", "phone" => 265, "symbol" => "MK", "capital" => "Lilongwe", "currency" => "MWK", "continent" => "Africa", "continent_code" => "AF"),
"MY" => array("name" => "Malaysia", "phone" => 60, "symbol" => "RM", "capital" => "Kuala Lumpur", "currency" => "MYR", "continent" => "Asia", "continent_code" => "AS"),
"MV" => array("name" => "Maldives", "phone" => 960, "symbol" => "Rf", "capital" => "Male", "currency" => "MVR", "continent" => "Asia", "continent_code" => "AS"),
"ML" => array("name" => "Mali", "phone" => 223, "symbol" => "CFA", "capital" => "Bamako", "currency" => "XOF", "continent" => "Africa", "continent_code" => "AF"),
"MT" => array("name" => "Malta", "phone" => 356, "symbol" => "€", "capital" => "Valletta", "currency" => "EUR", "continent" => "Europe", "continent_code" => "EU"),
"MH" => array("name" => "Marshall Islands", "phone" => 692, "symbol" => "$", "capital" => "Majuro", "currency" => "USD", "continent" => "Oceania", "continent_code" => "OC"),
"MQ" => array("name" => "Martinique", "phone" => 596, "symbol" => "€", "capital" => "Fort-de-France", "currency" => "EUR", "continent" => "North America", "continent_code" => "NA"),
"MR" => array("name" => "Mauritania", "phone" => 222, "symbol" => "MRU", "capital" => "Nouakchott", "currency" => "MRO", "continent" => "Africa", "continent_code" => "AF"),
"MU" => array("name" => "Mauritius", "phone" => 230, "symbol" => "₨", "capital" => "Port Louis", "currency" => "MUR", "continent" => "Africa", "continent_code" => "AF"),
"YT" => array("name" => "Mayotte", "phone" => 262, "symbol" => "€", "capital" => "Mamoudzou", "currency" => "EUR", "continent" => "Africa", "continent_code" => "AF"),
"MX" => array("name" => "Mexico", "phone" => 52, "symbol" => "$", "capital" => "Mexico City", "currency" => "MXN", "continent" => "North America", "continent_code" => "NA"),
"FM" => array("name" => "Micronesia, Federated States of", "phone" => 691, "symbol" => "$", "capital" => "Palikir", "currency" => "USD", "continent" => "Oceania", "continent_code" => "OC"),
"MD" => array("name" => "Moldova, Republic of", "phone" => 373, "symbol" => "L", "capital" => "Chisinau", "currency" => "MDL", "continent" => "Europe", "continent_code" => "EU"),
"MC" => array("name" => "Monaco", "phone" => 377, "symbol" => "€", "capital" => "Monaco", "currency" => "EUR", "continent" => "Europe", "continent_code" => "EU"),
"MN" => array("name" => "Mongolia", "phone" => 976, "symbol" => "₮", "capital" => "Ulan Bator", "currency" => "MNT", "continent" => "Asia", "continent_code" => "AS"),
"ME" => array("name" => "Montenegro", "phone" => 382, "symbol" => "€", "capital" => "Podgorica", "currency" => "EUR", "continent" => "Europe", "continent_code" => "EU"),
"MS" => array("name" => "Montserrat", "phone" => 1664, "symbol" => "$", "capital" => "Plymouth", "currency" => "XCD", "continent" => "North America", "continent_code" => "NA"),
"MA" => array("name" => "Morocco", "phone" => 212, "symbol" => "DH", "capital" => "Rabat", "currency" => "MAD", "continent" => "Africa", "continent_code" => "AF"),
"MZ" => array("name" => "Mozambique", "phone" => 258, "symbol" => "MT", "capital" => "Maputo", "currency" => "MZN", "continent" => "Africa", "continent_code" => "AF"),
"MM" => array("name" => "Myanmar", "phone" => 95, "symbol" => "K", "capital" => "Nay Pyi Taw", "currency" => "MMK", "continent" => "Asia", "continent_code" => "AS"),
"NA" => array("name" => "Namibia", "phone" => 264, "symbol" => "$", "capital" => "Windhoek", "currency" => "NAD", "continent" => "Africa", "continent_code" => "AF"),
"NR" => array("name" => "Nauru", "phone" => 674, "symbol" => "$", "capital" => "Yaren", "currency" => "AUD", "continent" => "Oceania", "continent_code" => "OC"),
"NP" => array("name" => "Nepal", "phone" => 977, "symbol" => "₨", "capital" => "Kathmandu", "currency" => "NPR", "continent" => "Asia", "continent_code" => "AS"),
"NL" => array("name" => "Netherlands", "phone" => 31, "symbol" => "€", "capital" => "Amsterdam", "currency" => "EUR", "continent" => "Europe", "continent_code" => "EU"),
"AN" => array("name" => "Netherlands Antilles", "phone" => 599, "symbol" => "NAf", "capital" => "Willemstad", "currency" => "ANG", "continent" => "North America", "continent_code" => "NA"),
"NC" => array("name" => "New Caledonia", "phone" => 687, "symbol" => "₣", "capital" => "Noumea", "currency" => "XPF", "continent" => "Oceania", "continent_code" => "OC"),
"NZ" => array("name" => "New Zealand", "phone" => 64, "symbol" => "$", "capital" => "Wellington", "currency" => "NZD", "continent" => "Oceania", "continent_code" => "OC"),
"NI" => array("name" => "Nicaragua", "phone" => 505, "symbol" => "C$", "capital" => "Managua", "currency" => "NIO", "continent" => "North America", "continent_code" => "NA"),
"NE" => array("name" => "Niger", "phone" => 227, "symbol" => "CFA", "capital" => "Niamey", "currency" => "XOF", "continent" => "Africa", "continent_code" => "AF"),
"NG" => array("name" => "Nigeria", "phone" => 234, "symbol" => "₦", "capital" => "Abuja", "currency" => "NGN", "continent" => "Africa", "continent_code" => "AF"),
"NU" => array("name" => "Niue", "phone" => 683, "symbol" => "$", "capital" => "Alofi", "currency" => "NZD", "continent" => "Oceania", "continent_code" => "OC"),
"NF" => array("name" => "Norfolk Island", "phone" => 672, "symbol" => "$", "capital" => "Kingston", "currency" => "AUD", "continent" => "Oceania", "continent_code" => "OC"),
"MP" => array("name" => "Northern Mariana Islands", "phone" => 1670, "symbol" => "$", "capital" => "Saipan", "currency" => "USD", "continent" => "Oceania", "continent_code" => "OC"),
"NO" => array("name" => "Norway", "phone" => 47, "symbol" => "kr", "capital" => "Oslo", "currency" => "NOK", "continent" => "Europe", "continent_code" => "EU"),
"OM" => array("name" => "Oman", "phone" => 968, "symbol" => ".ع.ر", "capital" => "Muscat", "currency" => "OMR", "continent" => "Asia", "continent_code" => "AS"),
"PK" => array("name" => "Pakistan", "phone" => 92, "symbol" => "₨", "capital" => "Islamabad", "currency" => "PKR", "continent" => "Asia", "continent_code" => "AS"),
"PW" => array("name" => "Palau", "phone" => 680, "symbol" => "$", "capital" => "Melekeok", "currency" => "USD", "continent" => "Oceania", "continent_code" => "OC"),
"PS" => array("name" => "Palestinian Territory, Occupied", "phone" => 970, "symbol" => "₪", "capital" => "East Jerusalem", "currency" => "ILS", "continent" => "Asia", "continent_code" => "AS"),
"PA" => array("name" => "Panama", "phone" => 507, "symbol" => "B/.", "capital" => "Panama City", "currency" => "PAB", "continent" => "North America", "continent_code" => "NA"),
"PG" => array("name" => "Papua New Guinea", "phone" => 675, "symbol" => "K", "capital" => "Port Moresby", "currency" => "PGK", "continent" => "Oceania", "continent_code" => "OC"),
"PY" => array("name" => "Paraguay", "phone" => 595, "symbol" => "₲", "capital" => "Asuncion", "currency" => "PYG", "continent" => "South America", "continent_code" => "SA"),
"PE" => array("name" => "Peru", "phone" => 51, "symbol" => "S/.", "capital" => "Lima", "currency" => "PEN", "continent" => "South America", "continent_code" => "SA"),
"PH" => array("name" => "Philippines", "phone" => 63, "symbol" => "₱", "capital" => "Manila", "currency" => "PHP", "continent" => "Asia", "continent_code" => "AS"),
"PN" => array("name" => "Pitcairn", "phone" => 64, "symbol" => "$", "capital" => "Adamstown", "currency" => "NZD", "continent" => "Oceania", "continent_code" => "OC"),
"PL" => array("name" => "Poland", "phone" => 48, "symbol" => "zł", "capital" => "Warsaw", "currency" => "PLN", "continent" => "Europe", "continent_code" => "EU"),
"PT" => array("name" => "Portugal", "phone" => 351, "symbol" => "€", "capital" => "Lisbon", "currency" => "EUR", "continent" => "Europe", "continent_code" => "EU"),
"PR" => array("name" => "Puerto Rico", "phone" => 1787, "symbol" => "$", "capital" => "San Juan", "currency" => "USD", "continent" => "North America", "continent_code" => "NA"),
"QA" => array("name" => "Qatar", "phone" => 974, "symbol" => "ق.ر", "capital" => "Doha", "currency" => "QAR", "continent" => "Asia", "continent_code" => "AS"),
"RE" => array("name" => "Reunion", "phone" => 262, "symbol" => "€", "capital" => "Saint-Denis", "currency" => "EUR", "continent" => "Africa", "continent_code" => "AF"),
"RO" => array("name" => "Romania", "phone" => 40, "symbol" => "lei", "capital" => "Bucharest", "currency" => "RON", "continent" => "Europe", "continent_code" => "EU"),
"RU" => array("name" => "Russian Federation", "phone" => 7, "symbol" => "₽", "capital" => "Moscow", "currency" => "RUB", "continent" => "Asia", "continent_code" => "AS"),
"RW" => array("name" => "Rwanda", "phone" => 250, "symbol" => "FRw", "capital" => "Kigali", "currency" => "RWF", "continent" => "Africa", "continent_code" => "AF"),
"BL" => array("name" => "Saint Barthelemy", "phone" => 590, "symbol" => "€", "capital" => "Gustavia", "currency" => "EUR", "continent" => "North America", "continent_code" => "NA"),
"SH" => array("name" => "Saint Helena", "phone" => 290, "symbol" => "£", "capital" => "Jamestown", "currency" => "SHP", "continent" => "Africa", "continent_code" => "AF"),
"KN" => array("name" => "Saint Kitts and Nevis", "phone" => 1869, "symbol" => "$", "capital" => "Basseterre", "currency" => "XCD", "continent" => "North America", "continent_code" => "NA"),
"LC" => array("name" => "Saint Lucia", "phone" => 1758, "symbol" => "$", "capital" => "Castries", "currency" => "XCD", "continent" => "North America", "continent_code" => "NA"),
"MF" => array("name" => "Saint Martin", "phone" => 590, "symbol" => "€", "capital" => "Marigot", "currency" => "EUR", "continent" => "North America", "continent_code" => "NA"),
"PM" => array("name" => "Saint Pierre and Miquelon", "phone" => 508, "symbol" => "€", "capital" => "Saint-Pierre", "currency" => "EUR", "continent" => "North America", "continent_code" => "NA"),
"VC" => array("name" => "Saint Vincent and the Grenadines", "phone" => 1784, "symbol" => "$", "capital" => "Kingstown", "currency" => "XCD", "continent" => "North America", "continent_code" => "NA"),
"WS" => array("name" => "Samoa", "phone" => 684, "symbol" => "SAT", "capital" => "Apia", "currency" => "WST", "continent" => "Oceania", "continent_code" => "OC"),
"SM" => array("name" => "San Marino", "phone" => 378, "symbol" => "€", "capital" => "San Marino", "currency" => "EUR", "continent" => "Europe", "continent_code" => "EU"),
"ST" => array("name" => "Sao Tome and Principe", "phone" => 239, "symbol" => "Db", "capital" => "Sao Tome", "currency" => "STD", "continent" => "Africa", "continent_code" => "AF"),
"SA" => array("name" => "Saudi Arabia", "phone" => 966, "symbol" => "﷼", "capital" => "Riyadh", "currency" => "SAR", "continent" => "Asia", "continent_code" => "AS"),
"SN" => array("name" => "Senegal", "phone" => 221, "symbol" => "CFA", "capital" => "Dakar", "currency" => "XOF", "continent" => "Africa", "continent_code" => "AF"),
"RS" => array("name" => "Serbia", "phone" => 381, "symbol" => "din", "capital" => "Belgrade", "currency" => "RSD", "continent" => "Europe", "continent_code" => "EU"),
"CS" => array("name" => "Serbia and Montenegro", "phone" => 381, "symbol" => "din", "capital" => "Belgrade", "currency" => "RSD", "continent" => "Europe", "continent_code" => "EU"),
"SC" => array("name" => "Seychelles", "phone" => 248, "symbol" => "SRe", "capital" => "Victoria", "currency" => "SCR", "continent" => "Africa", "continent_code" => "AF"),
"SL" => array("name" => "Sierra Leone", "phone" => 232, "symbol" => "Le", "capital" => "Freetown", "currency" => "SLL", "continent" => "Africa", "continent_code" => "AF"),
"SG" => array("name" => "Singapore", "phone" => 65, "symbol" => "$", "capital" => "Singapur", "currency" => "SGD", "continent" => "Asia", "continent_code" => "AS"),
"SX" => array("name" => "St Martin", "phone" => 721, "symbol" => "ƒ", "capital" => "Philipsburg", "currency" => "ANG", "continent" => "North America", "continent_code" => "NA"),
"SK" => array("name" => "Slovakia", "phone" => 421, "symbol" => "€", "capital" => "Bratislava", "currency" => "EUR", "continent" => "Europe", "continent_code" => "EU"),
"SI" => array("name" => "Slovenia", "phone" => 386, "symbol" => "€", "capital" => "Ljubljana", "currency" => "EUR", "continent" => "Europe", "continent_code" => "EU"),
"SB" => array("name" => "Solomon Islands", "phone" => 677, "symbol" => "Si$", "capital" => "Honiara", "currency" => "SBD", "continent" => "Oceania", "continent_code" => "OC"),
"SO" => array("name" => "Somalia", "phone" => 252, "symbol" => "Sh.so.", "capital" => "Mogadishu", "currency" => "SOS", "continent" => "Africa", "continent_code" => "AF"),
"ZA" => array("name" => "South Africa", "phone" => 27, "symbol" => "R", "capital" => "Pretoria", "currency" => "ZAR", "continent" => "Africa", "continent_code" => "AF"),
"GS" => array("name" => "South Georgia and the South Sandwich Islands", "phone" => 500, "symbol" => "£", "capital" => "Grytviken", "currency" => "GBP", "continent" => "Antarctica", "continent_code" => "AN"),
"SS" => array("name" => "South Sudan", "phone" => 211, "symbol" => "£", "capital" => "Juba", "currency" => "SSP", "continent" => "Africa", "continent_code" => "AF"),
"ES" => array("name" => "Spain", "phone" => 34, "symbol" => "€", "capital" => "Madrid", "currency" => "EUR", "continent" => "Europe", "continent_code" => "EU"),
"LK" => array("name" => "Sri Lanka", "phone" => 94, "symbol" => "Rs", "capital" => "Colombo", "currency" => "LKR", "continent" => "Asia", "continent_code" => "AS"),
"SD" => array("name" => "Sudan", "phone" => 249, "symbol" => ".س.ج", "capital" => "Khartoum", "currency" => "SDG", "continent" => "Africa", "continent_code" => "AF"),
"SR" => array("name" => "Suriname", "phone" => 597, "symbol" => "$", "capital" => "Paramaribo", "currency" => "SRD", "continent" => "South America", "continent_code" => "SA"),
"SJ" => array("name" => "Svalbard and Jan Mayen", "phone" => 47, "symbol" => "kr", "capital" => "Longyearbyen", "currency" => "NOK", "continent" => "Europe", "continent_code" => "EU"),
"SZ" => array("name" => "Swaziland", "phone" => 268, "symbol" => "E", "capital" => "Mbabane", "currency" => "SZL", "continent" => "Africa", "continent_code" => "AF"),
"SE" => array("name" => "Sweden", "phone" => 46, "symbol" => "kr", "capital" => "Stockholm", "currency" => "SEK", "continent" => "Europe", "continent_code" => "EU"),
"CH" => array("name" => "Switzerland", "phone" => 41, "symbol" => "CHf", "capital" => "Berne", "currency" => "CHF", "continent" => "Europe", "continent_code" => "EU"),
"SY" => array("name" => "Syrian Arab Republic", "phone" => 963, "symbol" => "LS", "capital" => "Damascus", "currency" => "SYP", "continent" => "Asia", "continent_code" => "AS"),
"TW" => array("name" => "Taiwan, Province of China", "phone" => 886, "symbol" => "$", "capital" => "Taipei", "currency" => "TWD", "continent" => "Asia", "continent_code" => "AS"),
"TJ" => array("name" => "Tajikistan", "phone" => 992, "symbol" => "SM", "capital" => "Dushanbe", "currency" => "TJS", "continent" => "Asia", "continent_code" => "AS"),
"TZ" => array("name" => "Tanzania, United Republic of", "phone" => 255, "symbol" => "TSh", "capital" => "Dodoma", "currency" => "TZS", "continent" => "Africa", "continent_code" => "AF"),
"TH" => array("name" => "Thailand", "phone" => 66, "symbol" => "฿", "capital" => "Bangkok", "currency" => "THB", "continent" => "Asia", "continent_code" => "AS"),
"TL" => array("name" => "Timor-Leste", "phone" => 670, "symbol" => "$", "capital" => "Dili", "currency" => "USD", "continent" => "Asia", "continent_code" => "AS"),
"TG" => array("name" => "Togo", "phone" => 228, "symbol" => "CFA", "capital" => "Lome", "currency" => "XOF", "continent" => "Africa", "continent_code" => "AF"),
"TK" => array("name" => "Tokelau", "phone" => 690, "symbol" => "$", "capital" => "", "currency" => "NZD", "continent" => "Oceania", "continent_code" => "OC"),
"TO" => array("name" => "Tonga", "phone" => 676, "symbol" => "$", "capital" => "Nuku'alofa", "currency" => "TOP", "continent" => "Oceania", "continent_code" => "OC"),
"TT" => array("name" => "Trinidad and Tobago", "phone" => 1868, "symbol" => "$", "capital" => "Port of Spain", "currency" => "TTD", "continent" => "North America", "continent_code" => "NA"),
"TN" => array("name" => "Tunisia", "phone" => 216, "symbol" => "ت.د", "capital" => "Tunis", "currency" => "TND", "continent" => "Africa", "continent_code" => "AF"),
"TR" => array("name" => "Turkey", "phone" => 90, "symbol" => "₺", "capital" => "Ankara", "currency" => "TRY", "continent" => "Asia", "continent_code" => "AS"),
"TM" => array("name" => "Turkmenistan", "phone" => 7370, "symbol" => "T", "capital" => "Ashgabat", "currency" => "TMT", "continent" => "Asia", "continent_code" => "AS"),
"TC" => array("name" => "Turks and Caicos Islands", "phone" => 1649, "symbol" => "$", "capital" => "Cockburn Town", "currency" => "USD", "continent" => "North America", "continent_code" => "NA"),
"TV" => array("name" => "Tuvalu", "phone" => 688, "symbol" => "$", "capital" => "Funafuti", "currency" => "AUD", "continent" => "Oceania", "continent_code" => "OC"),
"UG" => array("name" => "Uganda", "phone" => 256, "symbol" => "USh", "capital" => "Kampala", "currency" => "UGX", "continent" => "Africa", "continent_code" => "AF"),
"UA" => array("name" => "Ukraine", "phone" => 380, "symbol" => "₴", "capital" => "Kiev", "currency" => "UAH", "continent" => "Europe", "continent_code" => "EU"),
"AE" => array("name" => "United Arab Emirates", "phone" => 971, "symbol" => "إ.د", "capital" => "Abu Dhabi", "currency" => "AED", "continent" => "Asia", "continent_code" => "AS"),
"GB" => array("name" => "United Kingdom", "phone" => 44, "symbol" => "£", "capital" => "London", "currency" => "GBP", "continent" => "Europe", "continent_code" => "EU"),
"US" => array("name" => "United States", "phone" => 1, "symbol" => "$", "capital" => "Washington", "currency" => "USD", "continent" => "North America", "continent_code" => "NA"),
"UM" => array("name" => "United States Minor Outlying Islands", "phone" => 1, "symbol" => "$", "capital" => "", "currency" => "USD", "continent" => "North America", "continent_code" => "NA"),
"UY" => array("name" => "Uruguay", "phone" => 598, "symbol" => "$", "capital" => "Montevideo", "currency" => "UYU", "continent" => "South America", "continent_code" => "SA"),
"UZ" => array("name" => "Uzbekistan", "phone" => 998, "symbol" => "лв", "capital" => "Tashkent", "currency" => "UZS", "continent" => "Asia", "continent_code" => "AS"),
"VU" => array("name" => "Vanuatu", "phone" => 678, "symbol" => "VT", "capital" => "Port Vila", "currency" => "VUV", "continent" => "Oceania", "continent_code" => "OC"),
"VE" => array("name" => "Venezuela", "phone" => 58, "symbol" => "Bs", "capital" => "Caracas", "currency" => "VEF", "continent" => "South America", "continent_code" => "SA"),
"VN" => array("name" => "Viet Nam", "phone" => 84, "symbol" => "₫", "capital" => "Hanoi", "currency" => "VND", "continent" => "Asia", "continent_code" => "AS"),
"VG" => array("name" => "Virgin Islands, British", "phone" => 1284, "symbol" => "$", "capital" => "Road Town", "currency" => "USD", "continent" => "North America", "continent_code" => "NA"),
"VI" => array("name" => "Virgin Islands, U.s.", "phone" => 1340, "symbol" => "$", "capital" => "Charlotte Amalie", "currency" => "USD", "continent" => "North America", "continent_code" => "NA"),
"WF" => array("name" => "Wallis and Futuna", "phone" => 681, "symbol" => "₣", "capital" => "Mata Utu", "currency" => "XPF", "continent" => "Oceania", "continent_code" => "OC"),
"EH" => array("name" => "Western Sahara", "phone" => 212, "symbol" => "MAD", "capital" => "El-Aaiun", "currency" => "MAD", "continent" => "Africa", "continent_code" => "AF"),
"YE" => array("name" => "Yemen", "phone" => 967, "symbol" => "﷼", "capital" => "Sanaa", "currency" => "YER", "continent" => "Asia", "continent_code" => "AS"),
"ZM" => array("name" => "Zambia", "phone" => 260, "symbol" => "ZK", "capital" => "Lusaka", "currency" => "ZMW", "continent" => "Africa", "continent_code" => "AF"),
"ZW" => array("name" => "Zimbabwe", "phone" => 263, "symbol" => "$", "capital" => "Harare", "currency" => "ZWL", "continent" => "Africa", "continent_code" => "AF")
);
}